12.02 Using conditional filtering routes
As an example, let's create a scenario that generates a random number between 0 and 20. The scenario will result in one of two messages:
- "The value is greater than 10" - if the generated number is greater than 10.
- "The value is less than or equal to 10" - if the generated number is 10 or less.
To set up this scenario, you need to add four nodes and two routes with filtering conditions:
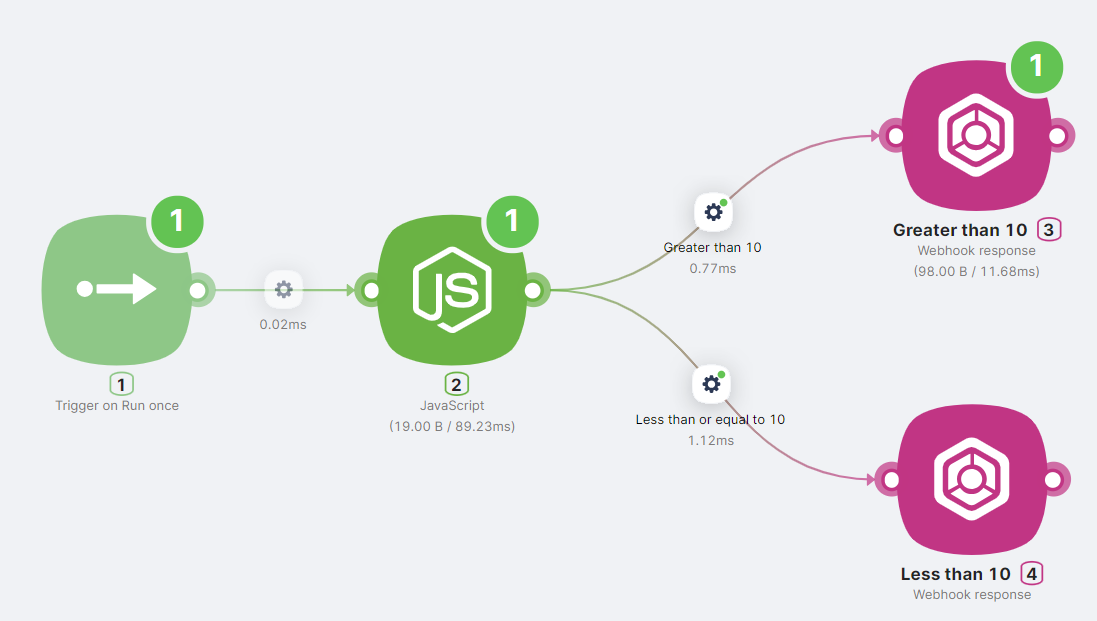
- (1) Trigger on Run once: This node initiates the scenario when you click the Run Once button;
- (2) JavaScript: This node contains code to generate a random number between 0 and 20;
export default async function run({execution_id, input, data, store}) {
const randomNumber = Math.floor(Math.random() * 20) + 1;
return {
randomNumber
}
}
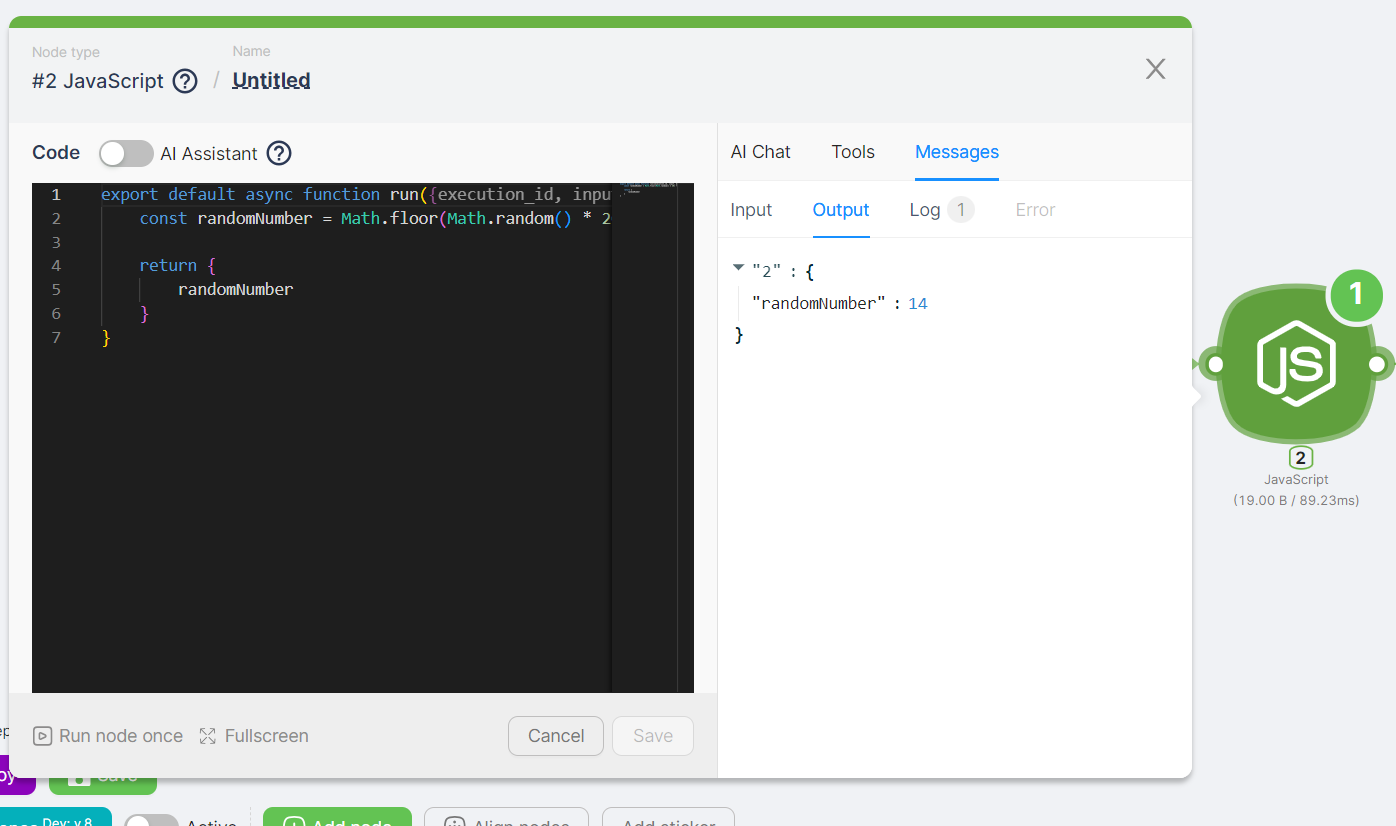
- (3) Webhook Response "Greater than 10": This node returns the message "The value is greater than 10";
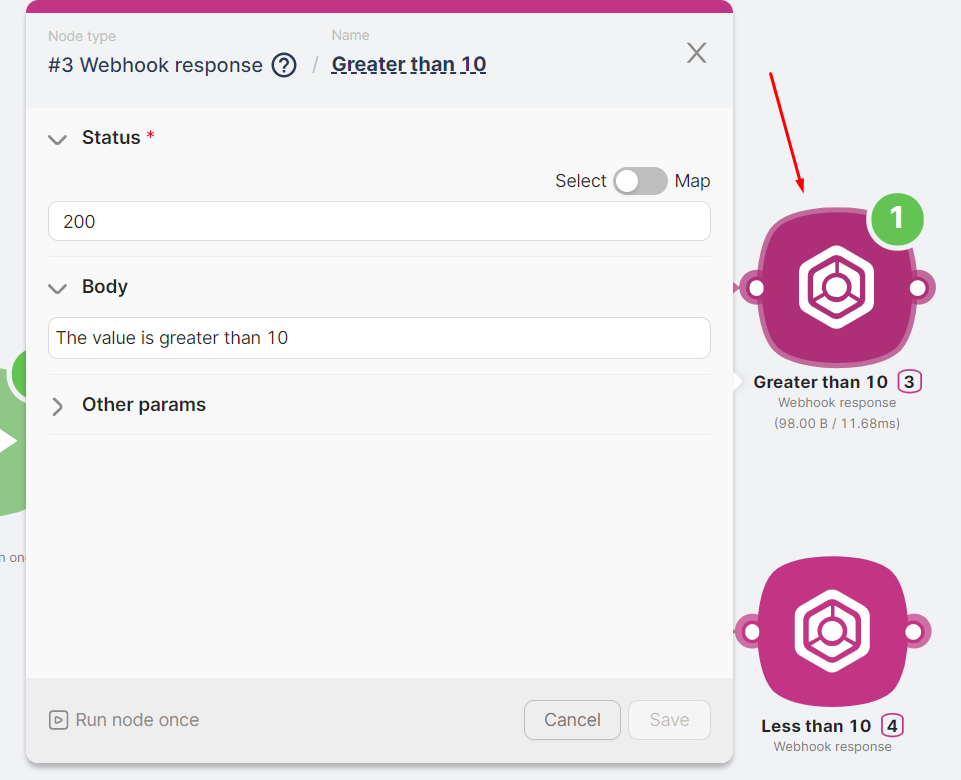
- (4) Route between JavaScript and Webhook Response "Greater than 10": This route has a filtering condition set to
{{$2.randomNumber > 10}}
;
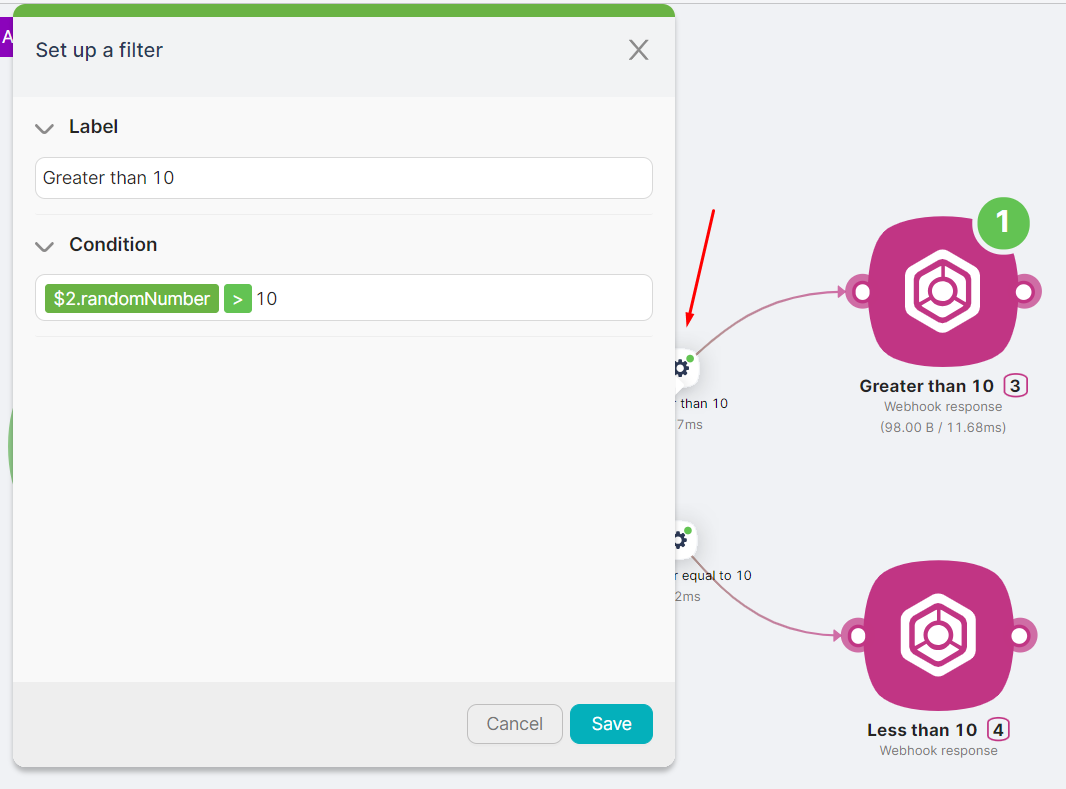
- (5) Webhook Response "Less than 10": This node returns the message "The value is less than or equal to 10";
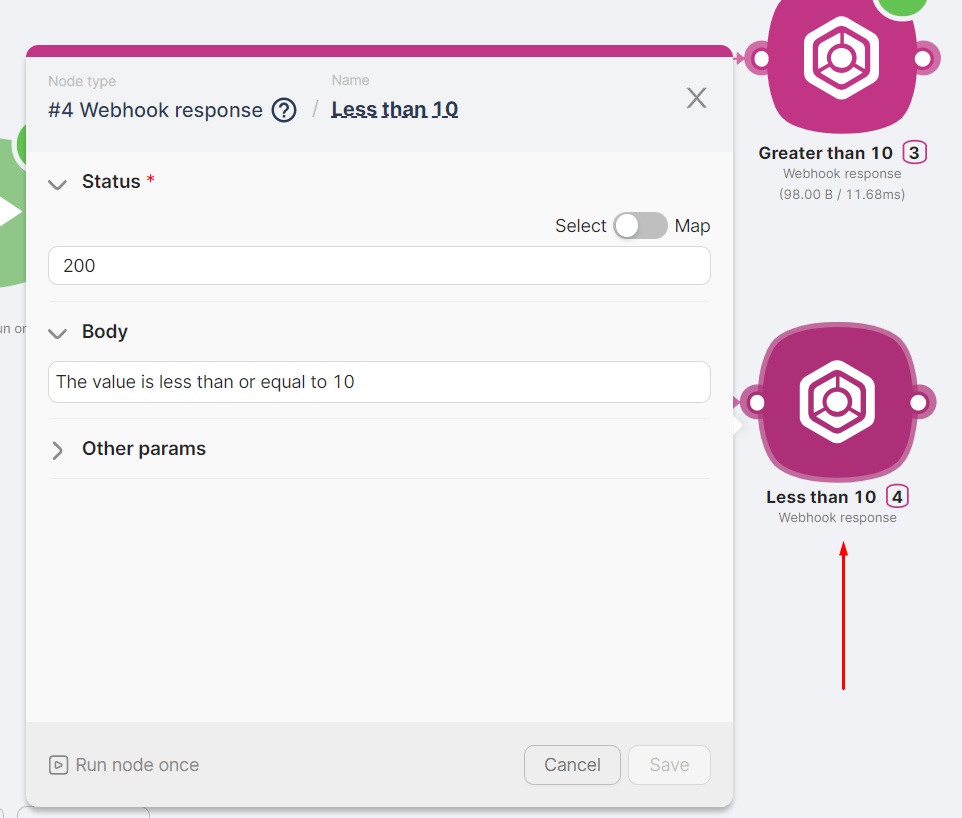
- (6) Route between JavaScript and Webhook Response "Less than 10": This route has a filtering condition set to
{{$2.randomNumber <= 10}}
;
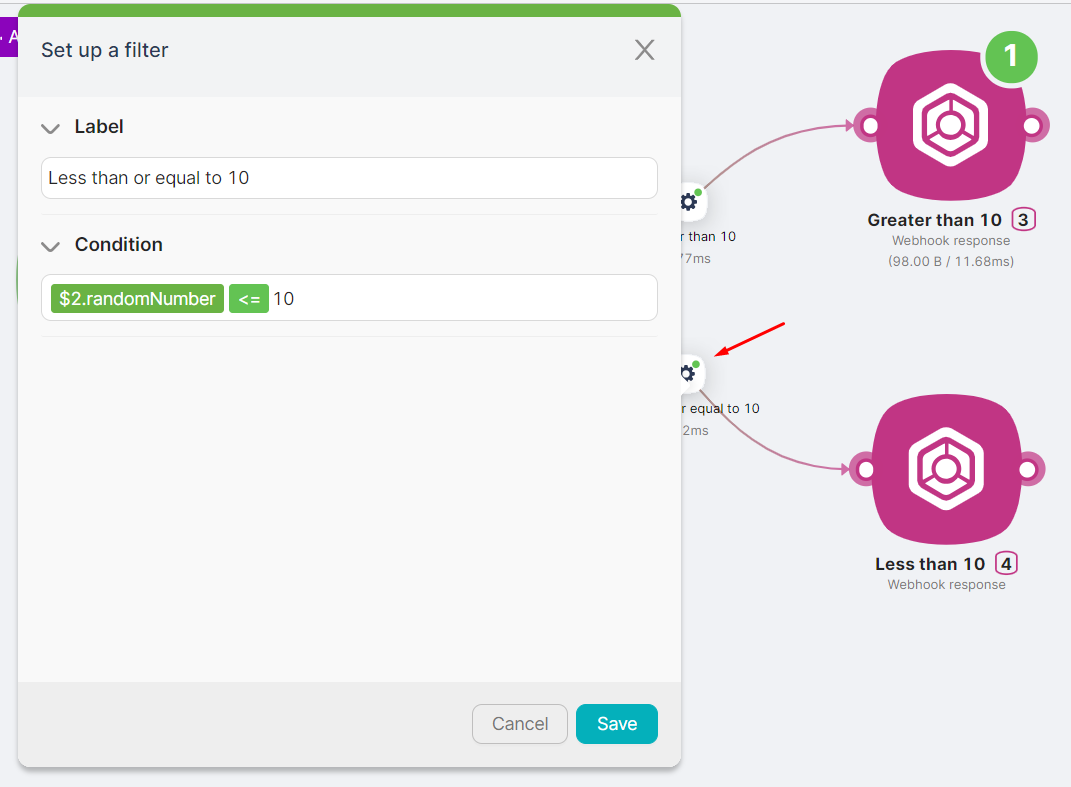
The result of the scenario is the appropriate message based on the generated value:
- If the generated number is 10 or less, the scenario follows the route "Less than or equal to 10", and the Webhook Response "Less than 10" node sends the response.
- If the generated number is greater than 10, the scenario follows the route "Greater than 10", and the Webhook Response "Greater than 10" node sends the response.