12.13 Using JS to manage global variables
As an example of using JavaScript to manage global variables, let's create a scenario in which:
- several global variables of different types are created;
- global variables created not today are deleted;
- a list of globally created variables today and numerical variables with values greater than 100 is returned;
- the value of a text variable is returned.
To successfully run the scenario, you need to add 6 nodes:

- (1) Trigger on Run once node to initiate a one-time execution of the script immediately after clicking the Run Once button;
- (2) JavaScript node named Create a GV is used to create global variables of different types with code:
export default async function run({ execution_id, input, data, store }) {
// Creating three numeric global variables
await store.setGlobalVariable("GlobalVar_number1", 100);
await store.setGlobalVariable("GlobalVar_number2", 200);
await store.setGlobalVariable("GlobalVar_number3", 300);
// Creating global variables with types of string and JSON
await store.setGlobalVariable("GlobalVarFromJs_string", "global var string value");
await store.setGlobalVariable("GlobalVarFromJs_obj", {"key":"global var object value"});
return {}; // Returning an empty object since no data needs to be returned
}
- (3) JavaScript node named Delete a GV is used to delete global variables created not today with code:
export default async function run({ execution_id, input, data, store }) {
// Get the list of global variables
const list = await store.listGlobalVariables();
// Get the current date in ISO format (without time)
const today = new Date().toISOString().slice(0, 10);
// Create an array of promises to delete variables that were not created today
const deletePromises = list
.filter(variable => {
// Convert the creation date of the variable to ISO format (without time)
const creationDate = new Date(variable.created_at).toISOString().slice(0, 10);
// Return true if the variable was not created today
return creationDate !== today;
})
.map(variable => {
// Delete the global variable that was not created today
return store.deleteGlobalVariable(variable.key);
});
// Execute all delete operations
await Promise.all(deletePromises);
// Return an empty object as the function should always return an object
return {
}
}
- (4) JavaScript node named List of GV is used to obtain a list of the remaining global variables, including numeric variables with values greater than 100, with code:
export default async function run({ execution_id, input, data, store }) {
// Retrieve the list of global variables
const list = await store.listGlobalVariables();
// Get the current date in ISO format (without time)
const today = new Date().toISOString().slice(0, 10);
// Create an array for numeric variables with a value greater than 100
const numericVariablesOver100 = list.filter(variable => {
return typeof variable.value === 'number' && variable.value > 100;
});
// Create an array for variables of other types, created today
const nonNumericVariablesCreatedToday = list.filter(variable => {
const creationDate = new Date(variable.created_at).toISOString().slice(0, 10);
return typeof variable.value !== 'number' && creationDate === today;
});
// Combine the two arrays
const variablesToReturn = [...numericVariablesOver100, ...nonNumericVariablesCreatedToday];
// Return the result
return {
variables: variablesToReturn
};
}
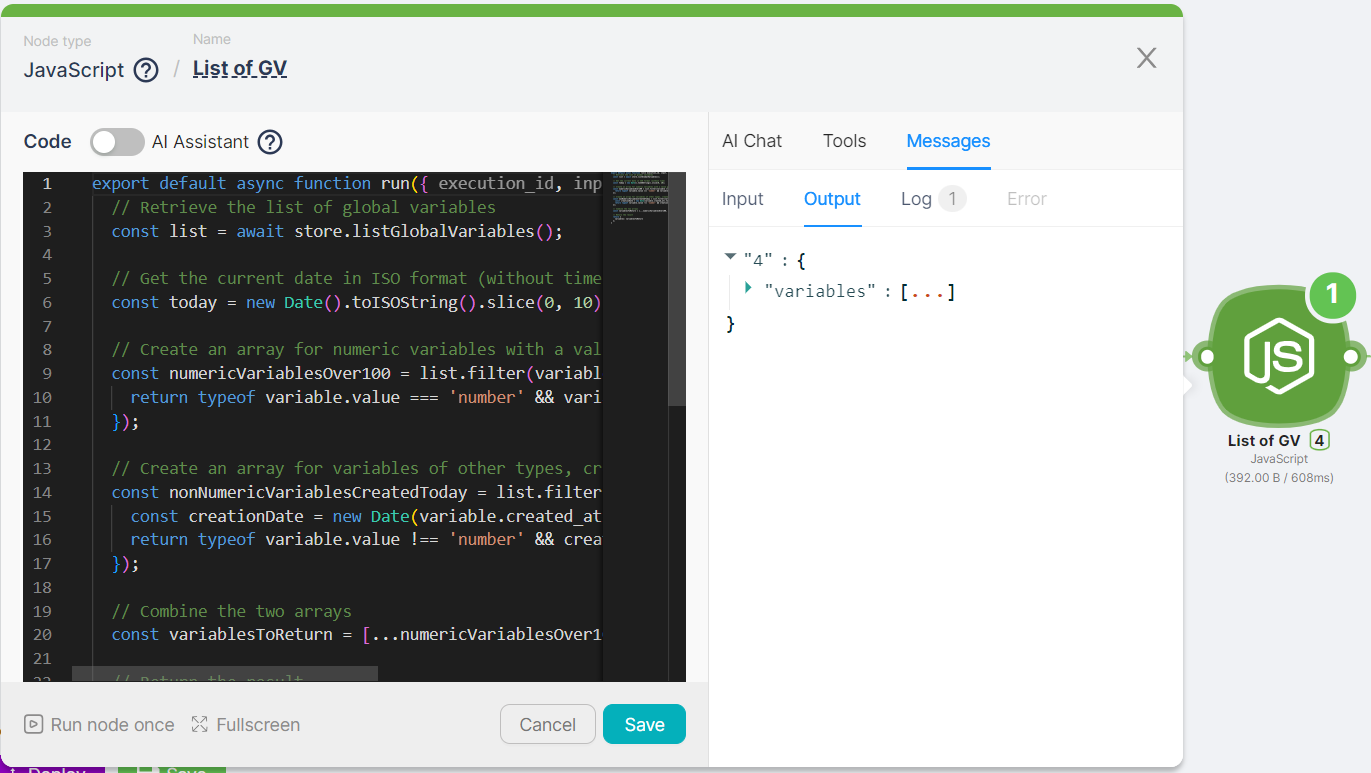
- (5) JavaScript node named Get a GV is used to obtain the value of a string global variable with code:
export default async function run({ execution_id, input, data, store }) {
// Get Global Var
const res_gv_str = await store.getGlobalVariable("GlobalVarFromJs_string")
return {
res_gv_str
}
}

- (6) Webhook response node to return the message "Ok" indicating the successful execution of the scenario.
As a result of the scenario, the output parameters of any of the JavaScript nodes and the execution of the specified actions with global variables. These parameters can be used if necessary in other scenarios or nodes.