03. Code
The Latenode platform allows you to use JavaScript to process data in the nodes of the Code group. Code execution in nodes takes place directly within the framework of the platform itself. This allows you to process data, apply various algorithms, or perform other operations directly inside the platform without having to switch to other tools or development environments.
Examples of possible code are available on this page.
In addition, some code usage options can be found on the Code group nodes page.
Libraries
The JavaScript node environment supports the import of npm libraries using the "import" statement. For example, importing and using the "lodash" library:
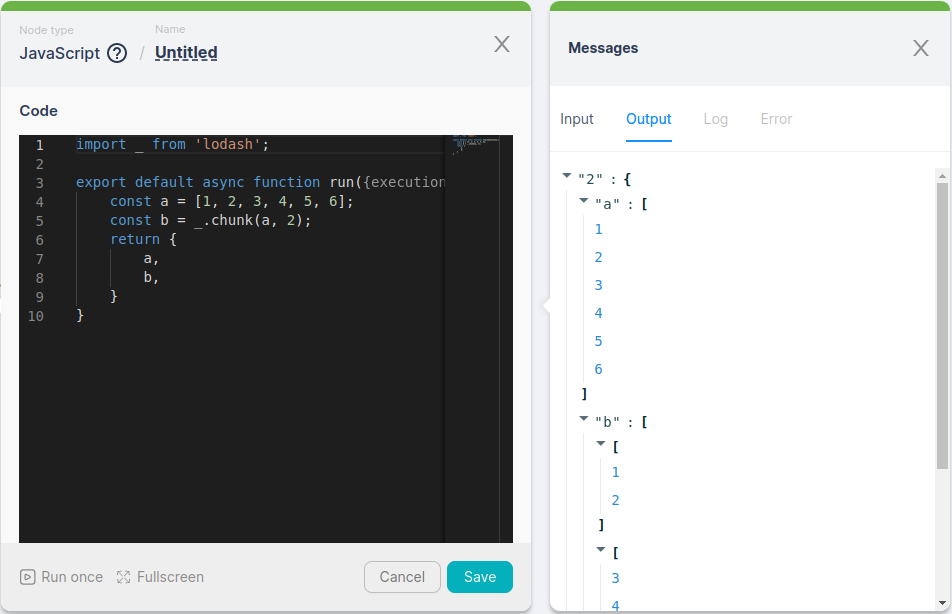
You can specify the version of the library you want to use using "@" symbol, for example:
import _ from '[email protected]';
import _ from 'axios@^1.2.0';
After each script with a JavaScript node is saved, a check is performed to see if there are any library imports and changes to the list of libraries and their versions (if specified):
- If there are changes, the libraries are installed.
- If there are no changes, the saved libraries and versions are used.
Library installation takes some time, and if the user starts the node before the installation is complete, they will receive an error message: "Dependency installation is not yet completed. Please try again in a few seconds." In this case, it is necessary to wait for a short while before proceeding.
Using NPM Packages
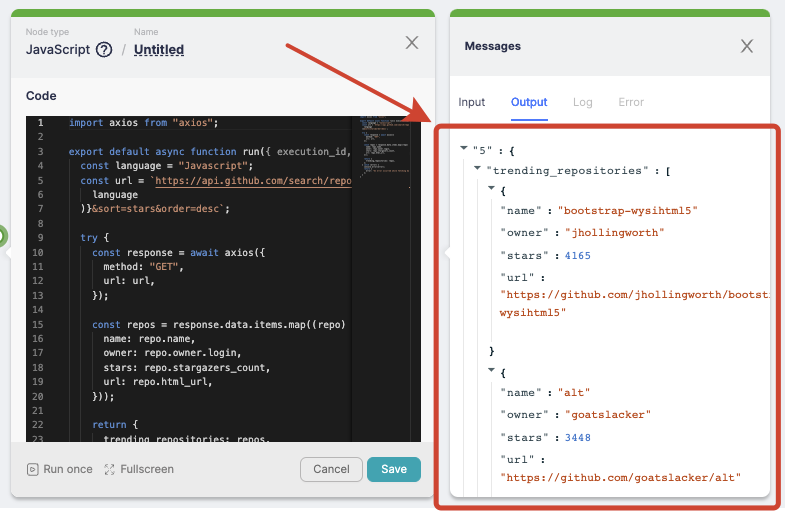
Node Package Manager (NPM) is a tool for developers working with Node.js, as it allows them to leverage a vast library of ready-made packages and easily manage project dependencies. Using the "axios" package enables developers to easily fetch data from external APIs or other web services without having to write extensive code for handling HTTP requests and responses.
An example of such a scenario is fetching a list of GitHub repositories based on a selected programming language using the Axios package
import axios from "axios";
export default async function run({ execution_id, input, data }) {
const language = "Javascript";
const url = \\`https://api.github.com/search/repositories?q=language:${encodeURIComponent(
language
)}&sort=stars&order=desc\\`;
try {
const response = await axios({
method: "GET",
url: url,
});
const repos = response.data.items.map((repo) => ({
name: repo.name,
owner: repo.owner.login,
stars: repo.stargazers_count,
url: repo.html_url,
}));
return {
trending_repositories: repos,
};
} catch (error) {
console.error(error);
return {
error: "An error occurred while fetching data from the GitHub API.",
};
}
}
Another example of using NPM packages is a script for calculating the time remaining until a deadline using the Moment package:
import moment from "moment";
export default async function run({ execution_id, input, data }) {
const deadline = "25.10.2024"; // Retrieve deadline from input
const now = moment(); // Get current time
const deadlineMoment = moment(deadline, "DD.MM.YYYY"); // Parse deadline string to Moment object using custom format
const remainingTime = deadlineMoment.from(now); // Calculate remaining time
return {
remainingTime
};
}
Logging
In the code nodes, you can perform logging using the console.log
command. Here's an example using the Headless browser node:
async function run({execution_id, input, data, page}) {
console.log('Hello World!');
return {
}
}
You can check the logging by manually running the node by clicking the Run Once button:
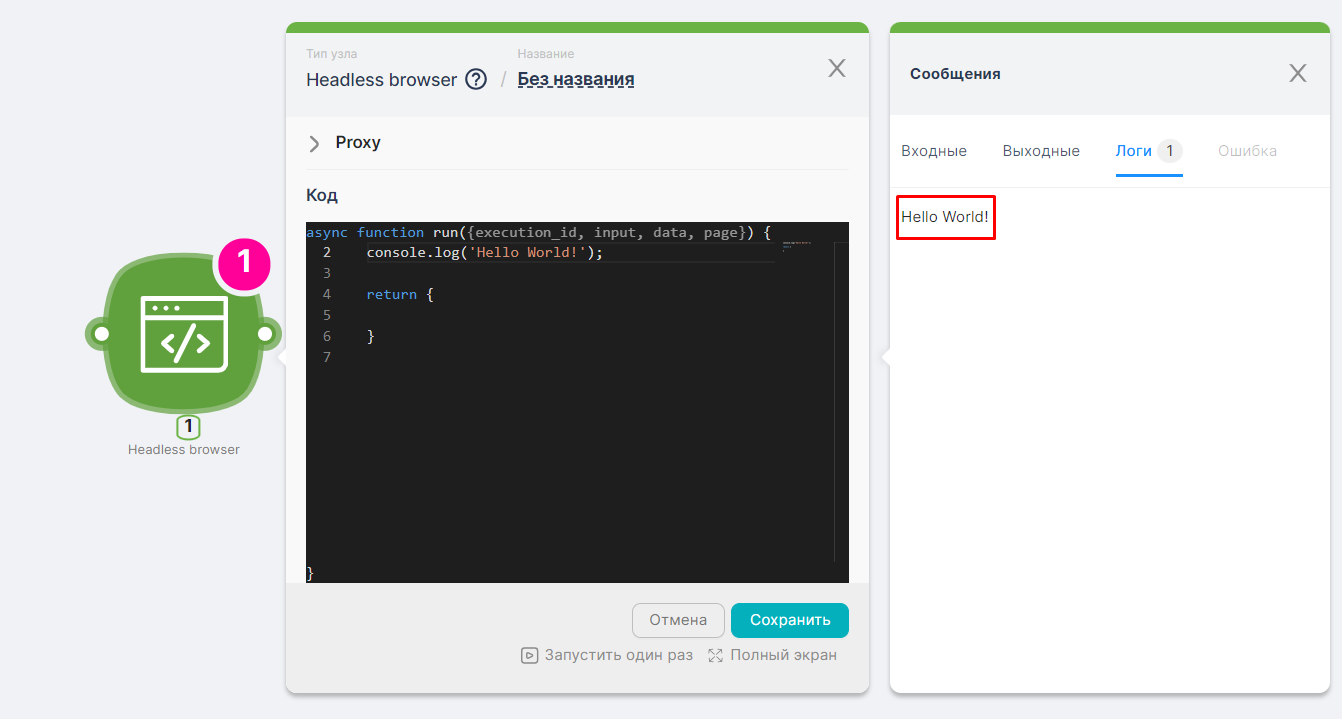
File Usage
Code nodes can be used to process files that have been sent, for example, using the POST method to a Trigger on Webhook node. Here is an example code snippet:
async function run({execution_id, input, data, page}) {
const file = data["{{2.body.files.[].content}}"];
}
In even when sending a single file, it is recognized as an array of files. Therefore, it is necessary to specify its index:
async function run({execution_id, input, data, page}) {
const file = data["{{2.body.files.[0].content}}"];
}
As a result, the file
constant will be assigned the path to a file, which can be used, for instance, to upload files into a particular form:
async function run({execution_id, input, data, page}) {
const file = data["{{2.body.files.[0].content}}"];
await (await page.$x('//*/input[@type="file"]'))[0].uploadFile(file);
}
Using an Array of Files
To iterate through an array of files with a known length, for example, 5, you need to write the following code:
async function run({execution_id, input, data, page}) {
const files = [
data["{{2.body.files.[0].content}}"],
data["{{2.body.files.[1].content}}"],
data["{{2.body.files.[2].content}}"],
data["{{2.body.files.[3].content}}"],
data["{{2.body.files.[4].content}}"]
].filter(file => file && file !== 'null');
const uploadForm = await page.$x('//*/input[@type="file"]')[0];
for (let file of files) {
await uploadForm.uploadFile(file);
}
}