12.07 Using the Add Multiple Rows node
To illustrate, let's create a scenario that results in transferring the representation of Airtable to a Google Sheet. The successful execution of the scenario requires adding 8 nodes.
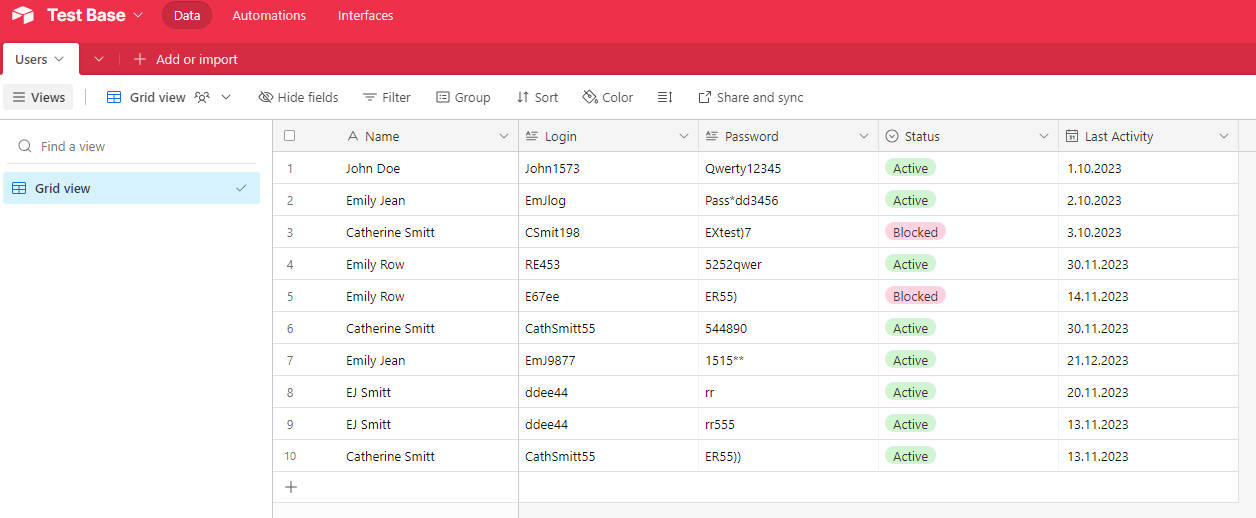
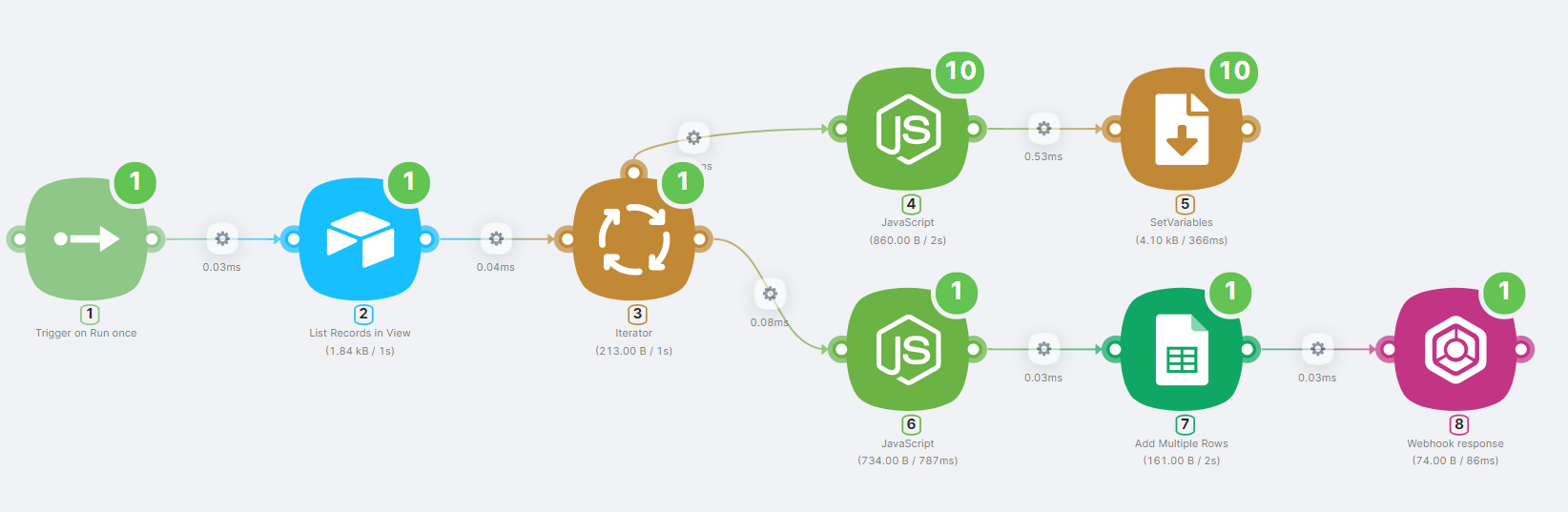
- (1) A Trigger on Run once node to initiate a single scenario run immediately after clicking the Run Once button;
- (2) A List Records in View node retrieves an array of rows from the selected view. To configure it correctly, establish a authorization, choose the relevant database, and view. The node outputs an array with information about each row. In addition to necessary data, row information includes the creation date and identifier;
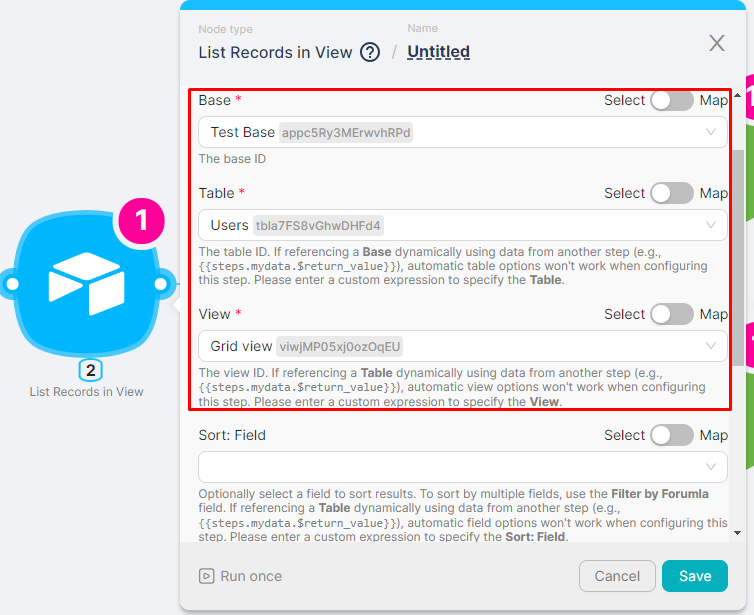
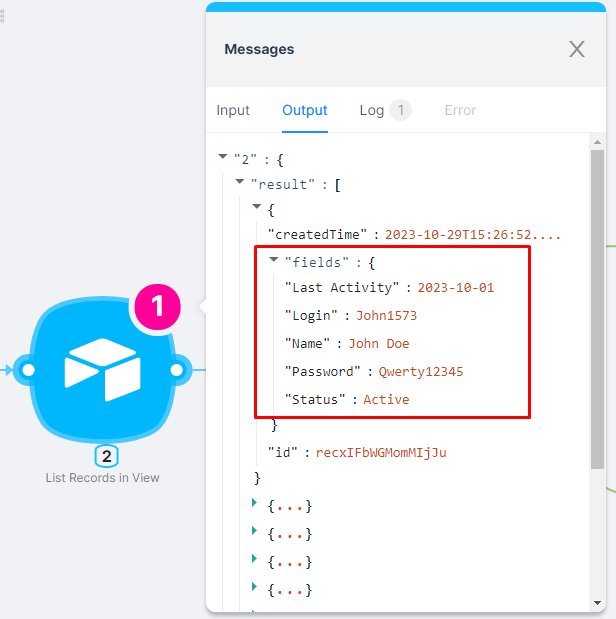
- (3) An Iterator node contains a sequence of values from the array of the List Records in View node;
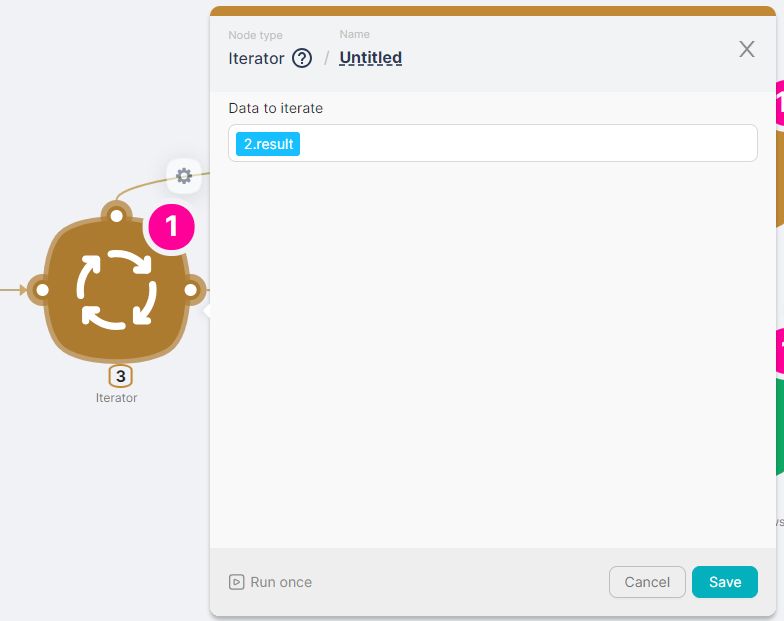
- (4) A first JavaScript node processes the received information about the view's row. During processing, the required information
data["{{3.value.fields}}"]
is transformed into an array of string values separated by commas.
export default async function run({execution_id, input, data}) {
// Parse the JSON string from the data of node 3 (Iterator)
let record = JSON.parse(data["{{3.value.fields}}"]);
// If the record exists, we transform it into an array of values, otherwise we return an empty array
let recordArray = record ? Object.values(record) : [];
// Create a new array
let newArray = [];
// We transform each value from recordArray into a string, wrap it in double quotes,
// join all the values into one string separated by commas, and add it to newArray
newArray.push(recordArray.map(value => \`"${value}"\`).join(", "));
// Return the new array
return {
newArray
}
}
The node's output is an array in the desired format:
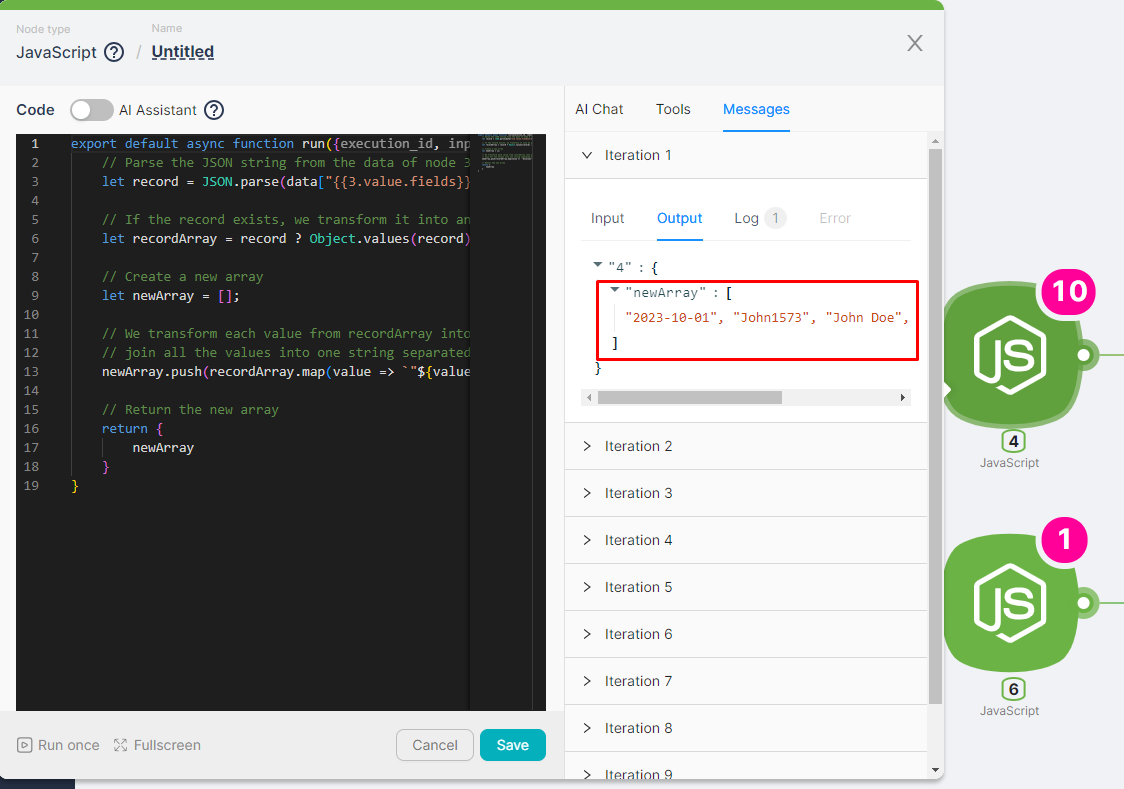
- (5) A SetVariables node constructs a single array from the multiple arrays output by the previous JavaScript node. The output consists of an array of arrays.
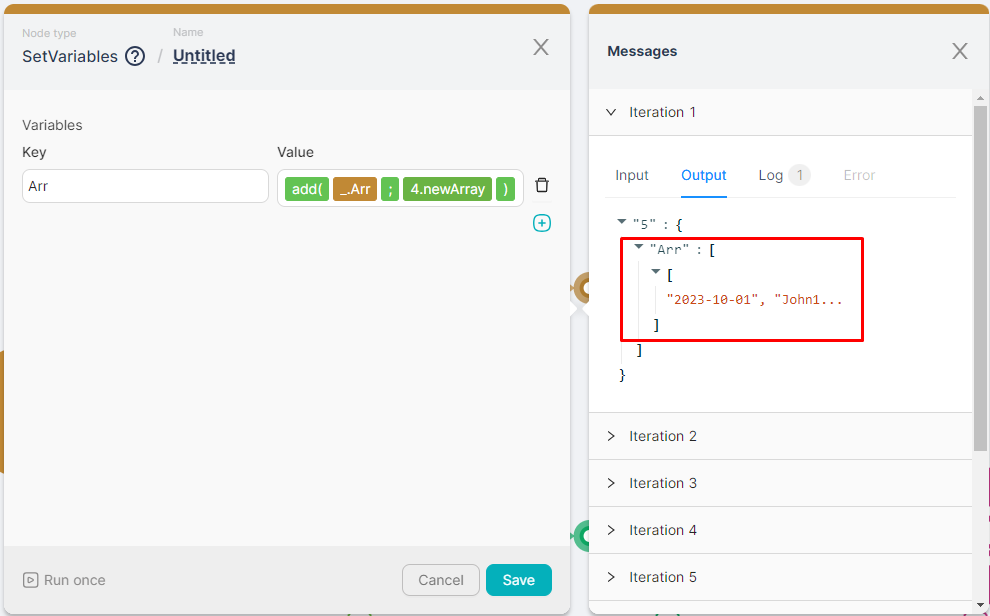
- (6) A second JavaScript node processes the array of arrays into the desired format with the following code:
export default async function run({execution_id, input, data}) {
// get the Arr array from data
const Arr = JSON.parse(data["{{_.Arr}}"]);
// convert the entire array to a string
let result = JSON.stringify(Arr);
// remove escape characters
result = result.replace(/\\/g, '');
// replace "" with "
result = result.replace(/""/g, '"');
return {
result: result
}
}
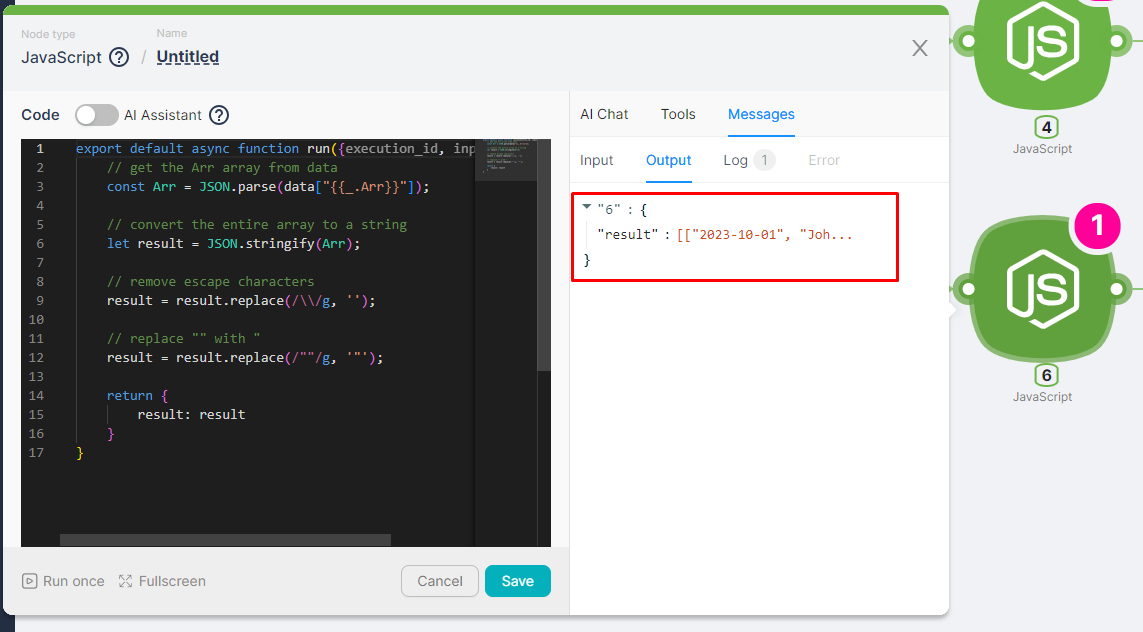
- An Add Multiple Rows node records the view's rows into a Google Sheet. To configure the node, set up the authorization. In the Row Values field, place the output of the previous JavaScript node;
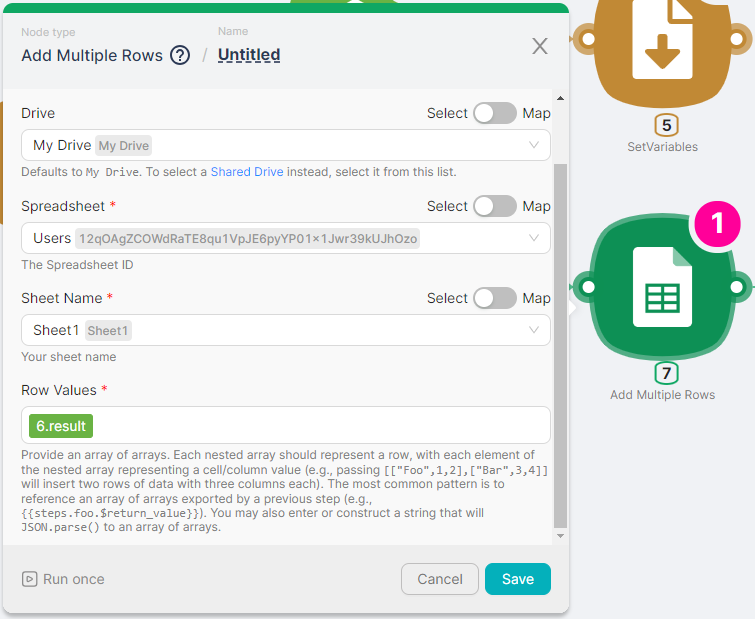
- (8) A Webhook Response node returns the response "Ok" upon successful execution of the scenario.
The result of executing the scenario is the copying of the selected view from the Airtable database to a Google Sheet.
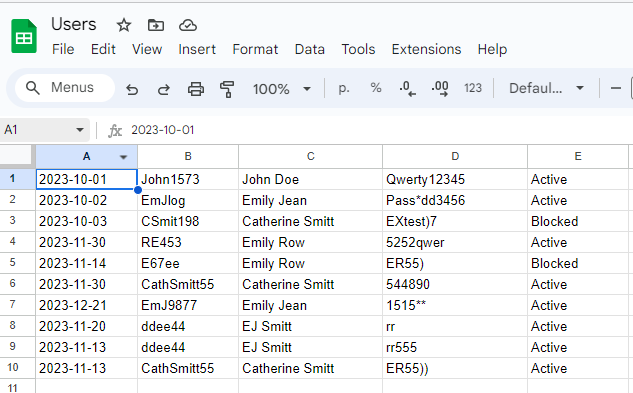